Ascio Web Service v3
C# CreateOrder
The THCH-entry will be auto-renewed. It will be renewed at the expire-date. Please use Expire to activate Auto-Expire.
Unexpire Mark
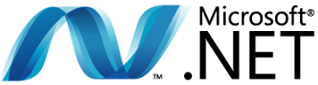
CreateOrderResponse CreateOrder(SecurityHeaderDetails securityHeader,CreateOrderRequest request)
Response codes
ResultCode | Message | Value |
---|---|---|
200 | OK | |
401 | Authorization failed | |
501 | Syntax error in parameters or arguments | |
506 | Required attribute missing in request |
CreateOrder Request
Property | Type |
---|---|
request |
CreateOrderResponse
Property | Description |
---|---|
CreateOrderResult | |
OrderInfo | |
ResultCode | Example: 1 |
ResultMessage | |
Errors |
Used in Classes
Property | Value |
request | MarkOrderRequest |
---|---|
OrderType | Unxpire |
C# example
AscioServices services = new ServiceReference1.AscioServiceClient();
SecurityHeaderDetails securityHeader = new SecurityHeaderDetails();
securityHeader.Account = "username";
securityHeader.Password = "password";
Registrant owner = new Registrant();
owner.FirstName = "John";
owner.FirstNameSpecified = true;
owner.LastName = "Doe";
owner.LastNameSpecified = true;
owner.OrgName = "Ascio";
owner.OrgNameSpecified = true;
owner.Address1 = "Address1Test";
owner.Address1Specified = true;
owner.Address2 = "Address2Test";
owner.Address2Specified = true;
owner.City = "CityTest";
owner.CitySpecified = true;
owner.State = "StateTest";
owner.StateSpecified = true;
owner.PostalCode = "888349";
owner.PostalCodeSpecified = true;
owner.CountryCode = "DK";
owner.CountryCodeSpecified = true;
owner.Phone = "+45.123456789";
owner.PhoneSpecified = true;
owner.Fax = "+45.987654321";
owner.FaxSpecified = true;
owner.Email = email@email.com
owner.Type = "owner";
owner.TypeSpecified = true;
owner.Details = "DetailsTest";
owner.DetailsSpecified = true;
owner.OrganisationNumber = "OrganisationNumberTest";
owner.OrganisationNumberSpecified = true;
owner.VatNumber = "VatNumberTest";
owner.VatNumberSpecified = true;
owner.NexusCategory = "NexusCategoryTest";
owner.NexusCategorySpecified = true;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions resellerExtensions = new Extensions({(
new KeyValue("Title", "Mrs.")
}
);
Contact reseller = new Contact();
reseller.FirstName = "John";
reseller.FirstNameSpecified = true;
reseller.LastName = "Doe";
reseller.LastNameSpecified = true;
reseller.OrgName = "Ascio";
reseller.OrgNameSpecified = true;
reseller.Address1 = "Address1Test";
reseller.Address1Specified = true;
reseller.Address2 = "Address2Test";
reseller.Address2Specified = true;
reseller.City = "CityTest";
reseller.CitySpecified = true;
reseller.State = "StateTest";
reseller.StateSpecified = true;
reseller.PostalCode = "888349";
reseller.PostalCodeSpecified = true;
reseller.CountryCode = "DK";
reseller.CountryCodeSpecified = true;
reseller.Phone = "+45.123456789";
reseller.PhoneSpecified = true;
reseller.Fax = "+45.987654321";
reseller.FaxSpecified = true;
reseller.Email = email@email.com
reseller.Type = "owner";
reseller.TypeSpecified = true;
reseller.Details = "DetailsTest";
reseller.DetailsSpecified = true;
reseller.OrganisationNumber = "OrganisationNumberTest";
reseller.OrganisationNumberSpecified = true;
reseller.Extensions = resellerExtensions;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions markExtensions = new Extensions(array($keyValue));
TreatyOrStatuteMark mark = new TreatyOrStatuteMark();
mark.Handle = "JD123";
mark.HandleSpecified = true;
mark.MarkName = "MarkNameTest";
mark.MarkNameSpecified = true;
mark.MarkId = "MarkIdTest";
mark.MarkIdSpecified = true;
mark.AuthInfo = "X4FF!zu";
mark.AuthInfoSpecified = true;
mark.ServiceType = MarkServiceType.Standard;
mark.ServiceTypeSpecified = true;
mark.GoodsAndServicesDescription = "GoodsAndServicesDescriptionTest";
mark.GoodsAndServicesDescriptionSpecified = true;
mark.Labels = array(
);
mark.ClaimEmailNotification1 = "ClaimEmailNotification1Test";
mark.ClaimEmailNotification1Specified = true;
mark.ClaimEmailNotification2 = "ClaimEmailNotification2Test";
mark.ClaimEmailNotification2Specified = true;
mark.ClaimEmailNotification3 = "ClaimEmailNotification3Test";
mark.ClaimEmailNotification3Specified = true;
mark.ClaimEmailNotification4 = "ClaimEmailNotification4Test";
mark.ClaimEmailNotification4Specified = true;
mark.ClaimEmailNotification5 = "ClaimEmailNotification5Test";
mark.ClaimEmailNotification5Specified = true;
mark.NotificationFrequency = NotificationFrequencyType.Daily;
mark.NotificationFrequencySpecified = true;
mark.Owner = owner;
mark.Reseller = reseller;
mark.Extensions = markExtensions;
mark.ObjectComment = "Example Object Comment";
mark.ObjectCommentSpecified = true;
mark.Title = "TitleTest";
mark.TitleSpecified = true;
mark.ReferenceNumber = "ReferenceNumberTest";
mark.ReferenceNumberSpecified = true;
mark.Country = "DK";
mark.CountrySpecified = true;
mark.Region = "RegionTest";
mark.RegionSpecified = true;
mark.ProtectionDate = new \DateTime();
mark.ProtectionDateSpecified = true;
mark.ExecutionDate = new \DateTime();
mark.ExecutionDateSpecified = true;
MarkOrderDocument markOrderDocument = new MarkOrderDocument();
markOrderDocument.FileName = "anything.jpg";
markOrderDocument.FileNameSpecified = true;
markOrderDocument.Content = base64_encode(123);
markOrderDocument.ContentSpecified = true;
markOrderDocument.DocType = MarkOrderDocType.TrademarkAssigneeDeclaration;
markOrderDocument.DocTypeSpecified = true;
ArrayOfMarkOrderDocument documents = new ArrayOfMarkOrderDocument(array($markOrderDocument));
MarkOrderRequest request = new MarkOrderRequest();
request.Type = OrderType.Register;
request.TypeSpecified = true;
request.Period = 1;
request.PeriodSpecified = true;
request.TransactionComment = "TransactionCommentTest";
request.TransactionCommentSpecified = true;
request.Comments = "RegistrarTag";
request.CommentsSpecified = true;
request.Documentation = "DocumentationTest";
request.DocumentationSpecified = true;
request.Options = "OptionsTest";
request.OptionsSpecified = true;
request.Mark = mark;
request.Documents = documents;
CreateOrderResponse response = services.CreateOrder(securityHeader, request);
if (response.ResultCode == 200) {
Console.WriteLine("My new orderId is : " + request.OrderInfo.OrderId);
} else {
if (response.Errors != null) {
Console.WriteLine(String.Join(",", response.Errors));
}
Console.WriteLine(response.ResultMessage)
}
WSDL for AWS v3
https://aws.demo.ascio.com/v3/aws.wsdl (OTE)
https://aws.ascio.com/v3/aws.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.
https://aws.demo.ascio.com/v3/aws.wsdl (OTE)
https://aws.ascio.com/v3/aws.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.