Ascio Web Service v3
C# CreateOrder - Register
Registers a domain. Please look at the TLD-Kit for special rules.
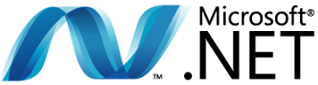
CreateOrderResponse CreateOrder(SecurityHeaderDetails securityHeader,CreateOrderRequest request)
Response codes
ResultCode | Message | Value |
---|---|---|
200 | Order validated | |
200 | Order received | |
400 | Order not validated | Messages |
401 | Authorization failed | |
405 | Access denied |
CreateOrder Request
Property | Type |
---|---|
request |
CreateOrderResponse
Property | Description |
---|---|
CreateOrderResult | |
OrderInfo | |
ResultCode | Example: 1 |
ResultMessage | |
Errors |
Used in Classes
Property | Value |
request | DomainOrderRequest |
---|---|
OrderType | Register |
C# example
AscioServices services = new ServiceReference1.AscioServiceClient();
SecurityHeaderDetails securityHeader = new SecurityHeaderDetails();
securityHeader.Account = "username";
securityHeader.Password = "password";
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions ownerExtensions = new Extensions(array($keyValue));
Registrant owner = new Registrant();
owner.FirstName = "John";
owner.FirstNameSpecified = true;
owner.LastName = "Doe";
owner.LastNameSpecified = true;
owner.OrgName = "Ascio";
owner.OrgNameSpecified = true;
owner.Address1 = "Address1Test";
owner.Address1Specified = true;
owner.Address2 = "Address2Test";
owner.Address2Specified = true;
owner.City = "CityTest";
owner.CitySpecified = true;
owner.State = "StateTest";
owner.StateSpecified = true;
owner.PostalCode = "888349";
owner.PostalCodeSpecified = true;
owner.CountryCode = "DK";
owner.CountryCodeSpecified = true;
owner.Phone = "+45.123456789";
owner.PhoneSpecified = true;
owner.Fax = "+45.987654321";
owner.FaxSpecified = true;
owner.Email = email@email.com
owner.Type = "owner";
owner.TypeSpecified = true;
owner.Details = "DetailsTest";
owner.DetailsSpecified = true;
owner.OrganisationNumber = "OrganisationNumberTest";
owner.OrganisationNumberSpecified = true;
owner.Extensions = ownerExtensions;
owner.VatNumber = "VatNumberTest";
owner.VatNumberSpecified = true;
owner.NexusCategory = "NexusCategoryTest";
owner.NexusCategorySpecified = true;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions adminExtensions = new Extensions({(
new KeyValue("Title", "Mrs.")
}
);
Contact admin = new Contact();
admin.FirstName = "John";
admin.FirstNameSpecified = true;
admin.LastName = "Doe";
admin.LastNameSpecified = true;
admin.OrgName = "Ascio";
admin.OrgNameSpecified = true;
admin.Address1 = "Address1Test";
admin.Address1Specified = true;
admin.Address2 = "Address2Test";
admin.Address2Specified = true;
admin.City = "CityTest";
admin.CitySpecified = true;
admin.State = "StateTest";
admin.StateSpecified = true;
admin.PostalCode = "888349";
admin.PostalCodeSpecified = true;
admin.CountryCode = "DK";
admin.CountryCodeSpecified = true;
admin.Phone = "+45.123456789";
admin.PhoneSpecified = true;
admin.Fax = "+45.987654321";
admin.FaxSpecified = true;
admin.Email = email@email.com
admin.Type = "owner";
admin.TypeSpecified = true;
admin.Details = "DetailsTest";
admin.DetailsSpecified = true;
admin.OrganisationNumber = "OrganisationNumberTest";
admin.OrganisationNumberSpecified = true;
admin.Extensions = adminExtensions;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions techExtensions = new Extensions({(
new KeyValue("Title", "Mrs.")
}
);
Contact tech = new Contact();
tech.FirstName = "John";
tech.FirstNameSpecified = true;
tech.LastName = "Doe";
tech.LastNameSpecified = true;
tech.OrgName = "Ascio";
tech.OrgNameSpecified = true;
tech.Address1 = "Address1Test";
tech.Address1Specified = true;
tech.Address2 = "Address2Test";
tech.Address2Specified = true;
tech.City = "CityTest";
tech.CitySpecified = true;
tech.State = "StateTest";
tech.StateSpecified = true;
tech.PostalCode = "888349";
tech.PostalCodeSpecified = true;
tech.CountryCode = "DK";
tech.CountryCodeSpecified = true;
tech.Phone = "+45.123456789";
tech.PhoneSpecified = true;
tech.Fax = "+45.987654321";
tech.FaxSpecified = true;
tech.Email = email@email.com
tech.Type = "owner";
tech.TypeSpecified = true;
tech.Details = "DetailsTest";
tech.DetailsSpecified = true;
tech.OrganisationNumber = "OrganisationNumberTest";
tech.OrganisationNumberSpecified = true;
tech.Extensions = techExtensions;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions billingExtensions = new Extensions({(
new KeyValue("Title", "Mrs.")
}
);
Contact billing = new Contact();
billing.FirstName = "John";
billing.FirstNameSpecified = true;
billing.LastName = "Doe";
billing.LastNameSpecified = true;
billing.OrgName = "Ascio";
billing.OrgNameSpecified = true;
billing.Address1 = "Address1Test";
billing.Address1Specified = true;
billing.Address2 = "Address2Test";
billing.Address2Specified = true;
billing.City = "CityTest";
billing.CitySpecified = true;
billing.State = "StateTest";
billing.StateSpecified = true;
billing.PostalCode = "888349";
billing.PostalCodeSpecified = true;
billing.CountryCode = "DK";
billing.CountryCodeSpecified = true;
billing.Phone = "+45.123456789";
billing.PhoneSpecified = true;
billing.Fax = "+45.987654321";
billing.FaxSpecified = true;
billing.Email = email@email.com
billing.Type = "owner";
billing.TypeSpecified = true;
billing.Details = "DetailsTest";
billing.DetailsSpecified = true;
billing.OrganisationNumber = "OrganisationNumberTest";
billing.OrganisationNumberSpecified = true;
billing.Extensions = billingExtensions;
NameServer nameServer1 = new NameServer();
nameServer1.Handle = "JD123";
nameServer1.HostName = "ns1.ascio.net";
nameServer1.IpAddress = "64.98.148.24";
nameServer1.IpV6Address = "xxxx:xxxx:xxx:xxx:xxxx";
nameServer1.Details = "DetailsTest";
NameServer nameServer2 = new NameServer();
nameServer2.Handle = "JD123";
nameServer2.HostName = "ns2.ascio.net";
nameServer2.IpAddress = "216.40.47.100";
nameServer2.IpV6Address = "xxxx:xxxx:xxx:xxx:xxxx";
nameServer2.Details = "DetailsTest";
NameServer nameServer3 = new NameServer();
nameServer3.Handle = "JD123";
nameServer3.HostName = "ns3.ascio.net";
nameServer3.IpAddress = "64.98.148.25";
nameServer3.IpV6Address = "xxxx:xxxx:xxx:xxx:xxxx";
nameServer3.Details = "DetailsTest";
NameServer nameServer4 = new NameServer();
nameServer4.Handle = "JD123";
nameServer4.HostName = "ns4.ascio.net";
nameServer4.IpAddress = "216.40.47.101";
nameServer4.IpV6Address = "xxxx:xxxx:xxx:xxx:xxxx";
nameServer4.Details = "DetailsTest";
NameServer nameServer5 = new NameServer();
nameServer5.Handle = "JD123";
nameServer5.HostName = "HostNameTest";
nameServer5.IpAddress = "IpAddressTest";
nameServer5.IpV6Address = "IpV6AddressTest";
nameServer5.Details = "DetailsTest";
NameServer nameServer6 = new NameServer();
nameServer6.Handle = "JD123";
nameServer6.HostName = "HostNameTest";
nameServer6.IpAddress = "IpAddressTest";
nameServer6.IpV6Address = "IpV6AddressTest";
nameServer6.Details = "DetailsTest";
NameServer nameServer7 = new NameServer();
nameServer7.Handle = "JD123";
nameServer7.HostName = "HostNameTest";
nameServer7.IpAddress = "IpAddressTest";
nameServer7.IpV6Address = "IpV6AddressTest";
nameServer7.Details = "DetailsTest";
NameServer nameServer8 = new NameServer();
nameServer8.Handle = "JD123";
nameServer8.HostName = "HostNameTest";
nameServer8.IpAddress = "IpAddressTest";
nameServer8.IpV6Address = "IpV6AddressTest";
nameServer8.Details = "DetailsTest";
NameServer nameServer9 = new NameServer();
nameServer9.Handle = "JD123";
nameServer9.HostName = "HostNameTest";
nameServer9.IpAddress = "IpAddressTest";
nameServer9.IpV6Address = "IpV6AddressTest";
nameServer9.Details = "DetailsTest";
NameServer nameServer10 = new NameServer();
nameServer10.Handle = "JD123";
nameServer10.HostName = "HostNameTest";
nameServer10.IpAddress = "IpAddressTest";
nameServer10.IpV6Address = "IpV6AddressTest";
nameServer10.Details = "DetailsTest";
NameServer nameServer11 = new NameServer();
nameServer11.Handle = "JD123";
nameServer11.HostName = "HostNameTest";
nameServer11.IpAddress = "IpAddressTest";
nameServer11.IpV6Address = "IpV6AddressTest";
nameServer11.Details = "DetailsTest";
NameServer nameServer12 = new NameServer();
nameServer12.Handle = "JD123";
nameServer12.HostName = "HostNameTest";
nameServer12.IpAddress = "IpAddressTest";
nameServer12.IpV6Address = "IpV6AddressTest";
nameServer12.Details = "DetailsTest";
NameServers nameServers = new NameServers();
nameServers.NameServer1 = nameServer1;
nameServers.NameServer2 = nameServer2;
nameServers.NameServer3 = nameServer3;
nameServers.NameServer4 = nameServer4;
nameServers.NameServer5 = nameServer5;
nameServers.NameServer6 = nameServer6;
nameServers.NameServer7 = nameServer7;
nameServers.NameServer8 = nameServer8;
nameServers.NameServer9 = nameServer9;
nameServers.NameServer10 = nameServer10;
nameServers.NameServer11 = nameServer11;
nameServers.NameServer12 = nameServer12;
DomainTradeMark trademark = new DomainTradeMark();
trademark.Name = "ascio-is-great.com";
trademark.Country = "DK";
trademark.Date = "2023-04-28T09:00:00";
trademark.Number = "NumberTest";
trademark.Type = "owner";
trademark.Contact = "ContactTest";
trademark.ContactLanguage = "ContactLanguageTest";
trademark.DocumentationLanguage = "DocumentationLanguageTest";
trademark.SecondContact = "SecondContactTest";
trademark.ThirdContact = "ThirdContactTest";
trademark.RegDate = "RegDateTest";
DnsSecKey dnsSecKey1 = new DnsSecKey();
dnsSecKey1.Handle = "JD123";
DnsSecKey dnsSecKey2 = new DnsSecKey();
dnsSecKey2.Handle = "JD123";
DnsSecKey dnsSecKey3 = new DnsSecKey();
dnsSecKey3.Handle = "JD123";
DnsSecKey dnsSecKey4 = new DnsSecKey();
dnsSecKey4.Handle = "JD123";
DnsSecKey dnsSecKey5 = new DnsSecKey();
dnsSecKey5.Handle = "JD123";
DnsSecKeys dnsSecKeys = new DnsSecKeys();
dnsSecKeys.DnsSecKey1 = dnsSecKey1;
dnsSecKeys.DnsSecKey2 = dnsSecKey2;
dnsSecKeys.DnsSecKey3 = dnsSecKey3;
dnsSecKeys.DnsSecKey4 = dnsSecKey4;
dnsSecKeys.DnsSecKey5 = dnsSecKey5;
KeyValue keyValue = new KeyValue();
keyValue.Key = "Title";
keyValue.KeySpecified = true;
keyValue.Value = "Mr.";
keyValue.ValueSpecified = true;
Extensions privacyProxyExtensions = new Extensions(array($keyValue));
PrivacyProxy privacyProxy = new PrivacyProxy();
privacyProxy.Type = PrivacyProxyType.None;
privacyProxy.PrivacyAdmin = false;
privacyProxy.PrivacyTech = false;
privacyProxy.PrivacyBilling = false;
privacyProxy.Extensions = privacyProxyExtensions;
Domain domain = new Domain();
domain.Name = "ascio-is-great.com";
domain.RenewPeriod = 1;
domain.DomainPurpose = "DomainPurposeTest";
domain.TransferLock = "Unlock";
domain.DeleteLock = "Unlock";
domain.UpdateLock = "Unlock";
domain.Owner = owner;
domain.Admin = admin;
domain.Tech = tech;
domain.Billing = billing;
domain.NameServers = nameServers;
domain.Trademark = trademark;
domain.DnsSecKeys = dnsSecKeys;
domain.PrivacyProxy = privacyProxy;
domain.DiscloseSocialData = "true";
domain.LocalPresence = false;
domain.MasterNameServerIp = "MasterNameServerIpTest";
DomainOrderRequest request = new DomainOrderRequest();
request.Type = OrderType.Register;
request.TypeSpecified = true;
request.TransactionComment = "TransactionCommentTest";
request.TransactionCommentSpecified = true;
request.Domain = domain;
request.AgreedPrice = 5000;
CreateOrderResponse response = services.CreateOrder(securityHeader, request);
if (response.ResultCode == 200) {
Console.WriteLine("My new orderId is : " + request.OrderInfo.OrderId);
} else {
if (response.Errors != null) {
Console.WriteLine(String.Join(",", response.Errors));
}
Console.WriteLine(response.ResultMessage)
}
WSDL for AWS v3
https://aws.demo.ascio.com/v3/aws.wsdl (OTE)
https://aws.ascio.com/v3/aws.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.
https://aws.demo.ascio.com/v3/aws.wsdl (OTE)
https://aws.ascio.com/v3/aws.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.