Ascio Web Service v2
Java Axis CreateOrder - Transfer_Domain
Registers a domain. Please look at the TLD-Kit for special rules. For some TLDs no registrant,contacts and nameservers are needed: com, net, org, biz, info, us, cc, cn, com.cn, net.cn, org.cn, tv.
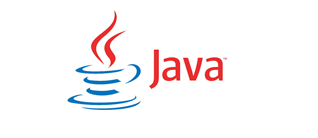
Response CreateOrder(string sessionId,Order order)
Response codes
ResultCode | Message | Value |
---|---|---|
200 | Order validated | |
200 | Order received | |
400 | Order not validated | Messages |
401 | Authorization failed | |
405 | Access denied |
CreateOrder Request
Property | Description |
---|---|
sessionId | Example: o58t9fjgw9bjarp6q7byv13e |
order |
CreateOrderResponse
Property |
---|
CreateOrderResult |
order |
Property | Value |
OrderType | Transfer_Domain |
---|
Java Axis example
package ascio.v2.examples;
import java.util.Calendar;
import com.ascio.www._2007._01.*;
import com.ascio.www._2007._01.holders.*;
import ascio.lib.*;
public class Transfer_DomainExample {
public static Response main() {
AscioConfig config = new AscioConfig();
V2 env = config.getTesting().getV2();
Registrant registrant = new Registrant();
registrant.setName("ascio-is-great.com");
registrant.setOrgName("Ascio");
registrant.setAddress1("Address1Test");
registrant.setAddress2("Address2Test");
registrant.setCity("CityTest");
registrant.setState("StateTest");
registrant.setPostalCode("888349");
registrant.setCountryCode("DK");
registrant.setEmail(config.getEmail());
registrant.setPhone("+45.123456789");
registrant.setFax("+45.987654321");
registrant.setRegistrantType("C1");
registrant.setVatNumber("VatNumberTest");
registrant.setNexusCategory("NexusCategoryTest");
registrant.setRegistrantNumber("abc123445");
registrant.setDetails("DetailsTest");
Please enter firstname [space] lastname, or lastname, firstname as value. The name is part of social data. You need an owner-chage to change the name. The Name is required for every Registrant/Contact. (In case no handle is used)The OrgName is part of social data. You need an owner-chage to change the OrgName.The Address1 is required for every Registrant/Contact. (In case no handle is used)The City is required for every Registrant/Contact. (In case no handle is used)The PostalCode is required for every Registrant/Contact. (In case no handle is used)The ISO 3166-1 code for the Country (DE,GB,DK,CH,SE,...). The CountryCode is required for every Registrant/Contact. (In case no handle is used)The Email is required for every Registrant/Contact. (In case no handle is used)Phone number must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.).Fax number must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.)Some TLDs need a Registrant Type. In most cases this is used to specify which type of RegistrantNumber is used (e.G. VAT, Passboard ID)An ID of the Registrant. The RegistrantType may describe which type of ID is used.
Contact adminContact = new Contact();
adminContact.setStatus("StatusTest");
adminContact.setHandle("JD123");
adminContact.setFirstName("John");
adminContact.setLastName("Doe");
adminContact.setOrgName("Ascio");
adminContact.setAddress1("Address1Test");
adminContact.setAddress2("Address2Test");
adminContact.setPostalCode("888349");
adminContact.setCity("CityTest");
adminContact.setState("StateTest");
adminContact.setCountryCode("DK");
adminContact.setEmail(config.getEmail());
adminContact.setPhone("+45.123456789");
adminContact.setFax("+45.987654321");
adminContact.setType("owner");
adminContact.setDetails("DetailsTest");
adminContact.setOrganisationNumber("OrganisationNumberTest");
The Handle. Please don't use Handle and contact-data. The data won't be merged!All contacts have FirstName and LastName. This is different than Registrants.All contacts have FirstName and LastName. This is different than Registrants.The Address1 is required for every Registrant/Contact. (In case no handle is used)The PostalCode is required for every Registrant/Contact. (In case no handle is used).The City is required for every Registrant/Contact. (In case no handle is used)The ISO 3166-1 code for the Country (DE,GB,DK,CH,SE,...). The CountryCode is required for every Registrant/Contact. (In case no handle is used)The Email is required for every Registrant/Contact. (In case no handle is used)Phone numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.).Fax numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.)
Contact techContact = new Contact();
techContact.setStatus("StatusTest");
techContact.setHandle("JD123");
techContact.setFirstName("John");
techContact.setLastName("Doe");
techContact.setOrgName("Ascio");
techContact.setAddress1("Address1Test");
techContact.setAddress2("Address2Test");
techContact.setPostalCode("888349");
techContact.setCity("CityTest");
techContact.setState("StateTest");
techContact.setCountryCode("DK");
techContact.setEmail(config.getEmail());
techContact.setPhone("+45.123456789");
techContact.setFax("+45.987654321");
techContact.setType("owner");
techContact.setDetails("DetailsTest");
techContact.setOrganisationNumber("OrganisationNumberTest");
The Handle. Please don't use Handle and contact-data. The data won't be merged!All contacts have FirstName and LastName. This is different than Registrants.All contacts have FirstName and LastName. This is different than Registrants.The Address1 is required for every Registrant/Contact. (In case no handle is used)The PostalCode is required for every Registrant/Contact. (In case no handle is used).The City is required for every Registrant/Contact. (In case no handle is used)The ISO 3166-1 code for the Country (DE,GB,DK,CH,SE,...). The CountryCode is required for every Registrant/Contact. (In case no handle is used)The Email is required for every Registrant/Contact. (In case no handle is used)Phone numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.).Fax numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.)
Contact billingContact = new Contact();
billingContact.setStatus("StatusTest");
billingContact.setHandle("JD123");
billingContact.setFirstName("John");
billingContact.setLastName("Doe");
billingContact.setOrgName("Ascio");
billingContact.setAddress1("Address1Test");
billingContact.setAddress2("Address2Test");
billingContact.setPostalCode("888349");
billingContact.setCity("CityTest");
billingContact.setState("StateTest");
billingContact.setCountryCode("DK");
billingContact.setEmail(config.getEmail());
billingContact.setPhone("+45.123456789");
billingContact.setFax("+45.987654321");
billingContact.setType("owner");
billingContact.setDetails("DetailsTest");
billingContact.setOrganisationNumber("OrganisationNumberTest");
The Handle. Please don't use Handle and contact-data. The data won't be merged!All contacts have FirstName and LastName. This is different than Registrants.All contacts have FirstName and LastName. This is different than Registrants.The Address1 is required for every Registrant/Contact. (In case no handle is used)The PostalCode is required for every Registrant/Contact. (In case no handle is used).The City is required for every Registrant/Contact. (In case no handle is used)The ISO 3166-1 code for the Country (DE,GB,DK,CH,SE,...). The CountryCode is required for every Registrant/Contact. (In case no handle is used)The Email is required for every Registrant/Contact. (In case no handle is used)Phone numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.).Fax numbers must be in E164a format, eg. +45.123456789 (no spaces, hyphens, etc.)
NameServer nameServer1 = new NameServer();
nameServer1.setHostName("ns1.ascio.net");
nameServer1.setIpAddress("64.98.148.24");
nameServer1.setStatus("StatusTest");
nameServer1.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer1.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer2 = new NameServer();
nameServer2.setHostName("ns2.ascio.net");
nameServer2.setIpAddress("216.40.47.100");
nameServer2.setStatus("StatusTest");
nameServer2.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer2.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer3 = new NameServer();
nameServer3.setHostName("ns3.ascio.net");
nameServer3.setIpAddress("64.98.148.25");
nameServer3.setStatus("StatusTest");
nameServer3.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer3.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer4 = new NameServer();
nameServer4.setHostName("ns4.ascio.net");
nameServer4.setIpAddress("216.40.47.101");
nameServer4.setStatus("StatusTest");
nameServer4.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer4.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer5 = new NameServer();
nameServer5.setHostName("HostNameTest");
nameServer5.setIpAddress("IpAddressTest");
nameServer5.setStatus("StatusTest");
nameServer5.setIpV6Address("IpV6AddressTest");
nameServer5.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer6 = new NameServer();
nameServer6.setHostName("HostNameTest");
nameServer6.setIpAddress("IpAddressTest");
nameServer6.setStatus("StatusTest");
nameServer6.setIpV6Address("IpV6AddressTest");
nameServer6.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer7 = new NameServer();
nameServer7.setHostName("HostNameTest");
nameServer7.setIpAddress("IpAddressTest");
nameServer7.setStatus("StatusTest");
nameServer7.setIpV6Address("IpV6AddressTest");
nameServer7.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer8 = new NameServer();
nameServer8.setHostName("HostNameTest");
nameServer8.setIpAddress("IpAddressTest");
nameServer8.setStatus("StatusTest");
nameServer8.setIpV6Address("IpV6AddressTest");
nameServer8.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer9 = new NameServer();
nameServer9.setHostName("HostNameTest");
nameServer9.setIpAddress("IpAddressTest");
nameServer9.setStatus("StatusTest");
nameServer9.setIpV6Address("IpV6AddressTest");
nameServer9.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer10 = new NameServer();
nameServer10.setHostName("HostNameTest");
nameServer10.setIpAddress("IpAddressTest");
nameServer10.setStatus("StatusTest");
nameServer10.setIpV6Address("IpV6AddressTest");
nameServer10.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer11 = new NameServer();
nameServer11.setHostName("HostNameTest");
nameServer11.setIpAddress("IpAddressTest");
nameServer11.setStatus("StatusTest");
nameServer11.setIpV6Address("IpV6AddressTest");
nameServer11.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServer nameServer12 = new NameServer();
nameServer12.setHostName("HostNameTest");
nameServer12.setIpAddress("IpAddressTest");
nameServer12.setStatus("StatusTest");
nameServer12.setIpV6Address("IpV6AddressTest");
nameServer12.setDetails("DetailsTest");
For normal orders you only need the HostName. We will set the IP. Exceptions are, TLDs with a ZoneCheck (like de,it,fr). An if you want to set a glue-recordYou need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.You need an IP Address if the ZoneCheck is used or you want to set at glue record. If you want to set a glue-record for ns1.mynsdomain.com then mynsdomain.com must be at ascio.
NameServers nameServers = new NameServers();
nameServers.setNameServer1(nameServer1);
nameServers.setNameServer2(nameServer2);
nameServers.setNameServer3(nameServer3);
nameServers.setNameServer4(nameServer4);
nameServers.setNameServer5(nameServer5);
nameServers.setNameServer6(nameServer6);
nameServers.setNameServer7(nameServer7);
nameServers.setNameServer8(nameServer8);
nameServers.setNameServer9(nameServer9);
nameServers.setNameServer10(nameServer10);
nameServers.setNameServer11(nameServer11);
nameServers.setNameServer12(nameServer12);
TradeMark trademark = new TradeMark();
trademark.setName("ascio-is-great.com");
trademark.setCountry("DK");
trademark.setDate(Calendar.getInstance());
trademark.setNumber("NumberTest");
trademark.setType("owner");
trademark.setContact("ContactTest");
trademark.setContactLanguage("ContactLanguageTest");
trademark.setDocumentationLanguage("DocumentationLanguageTest");
trademark.setSecondContact("SecondContactTest");
trademark.setThirdContact("ThirdContactTest");
trademark.setRegDate(Calendar.getInstance());
DnsSecKey dnsSecKey1 = new DnsSecKey();
dnsSecKey1.setHandle("JD123");
DnsSecKey dnsSecKey2 = new DnsSecKey();
dnsSecKey2.setHandle("JD123");
DnsSecKey dnsSecKey3 = new DnsSecKey();
dnsSecKey3.setHandle("JD123");
DnsSecKey dnsSecKey4 = new DnsSecKey();
dnsSecKey4.setHandle("JD123");
DnsSecKey dnsSecKey5 = new DnsSecKey();
dnsSecKey5.setHandle("JD123");
DnsSecKeys dnsSecKeys = new DnsSecKeys();
dnsSecKeys.setDnsSecKey1(dnsSecKey1);
dnsSecKeys.setDnsSecKey2(dnsSecKey2);
dnsSecKeys.setDnsSecKey3(dnsSecKey3);
dnsSecKeys.setDnsSecKey4(dnsSecKey4);
dnsSecKeys.setDnsSecKey5(dnsSecKey5);
Extension extension = new Extension();
extension.setKey("Title");
extension.setValue("Mr.");
ExtensionsExtension extensions[] = new ExtensionsExtension[]{extension};
PrivacyProxy privacyProxy = new PrivacyProxy();
privacyProxy.setType(PrivacyProxyType.None);
privacyProxy.setPrivacyAdmin(false);
privacyProxy.setPrivacyTech(false);
privacyProxy.setPrivacyBilling(false);
privacyProxy.setExtensions(extensions);
There are 2 types of proxy: Privacy: Only the e-mail address is replaced. Proxy: Everything is replaced with proxy data.The admin e-mail is replaced with a generated e-mail. In case of proxy all data is replaced.The tech e-mail is replaced with a generated e-mail. In case of proxy all data is replaced.The billing e-mail is replaced with a generated e-mail. In case of proxy all data is replaced.
Domain domain = new Domain();
domain.setDomainName(env.getProperties().getProperty("DomainName"));
domain.setAuthInfo("X4FF!zu");
domain.setRegistrant(registrant);
domain.setAdminContact(adminContact);
domain.setTechContact(techContact);
domain.setBillingContact(billingContact);
domain.setNameServers(nameServers);
domain.setTrademark(trademark);
domain.setDnsSecKeys(dnsSecKeys);
domain.setPrivacyProxy(privacyProxy);
domain.setDiscloseSocialData("true");
When you do GetDomain the AuthCode is in this parameter. We don't have a GetAuthCode method, please use GetDomain.The registrant is different from all other contacts. You can use a Registrant as Admin/Tech/Billing/Reseller contact. You can't you a Contact as Registrant
Order order = new Order();
order.setType(OrderType.Transfer_Domain);
order.setAccountReference("AccountReferenceTest");
order.setTransactionComment("TransactionCommentTest");
order.setComments("RegistrarTag");
order.setOptions("OptionsTest");
order.setLocalPresence("off");
order.setBatch("BatchTest");
order.setDocumentation("DocumentationTest");
order.setDomain(domain);
order.setAgreedPrice(5000);
Here partners can add their own data, like customer-id.Here partners can add their own comments, like customer-id.Referenced in the TLD-Kit as: 1cAscio offers many localpresences for countries with local-restrictions. Possible values are: LocalPresenceAdmin, LocalPresenceRegistrant,LocalPresenceAddresss RepresentativeThis field is deprecated. Please don't use it anymore.Please add your documentation ID herePremium prices are returned by the AvailabilityInfo. Please enter Premium price here, as confirmation that it is accepted.
try {
/**inputs holders*/
OrderHolder orderHolder = new OrderHolder(order);
Response response = env.getClient().createOrder(env.getSessionId(), orderHolder);
System.out.println("CreateOrder ResultCode\t: " + response.getResultCode().toString());
System.out.println("CreateOrder Message\t: " + response.getMessage());
System.out.println("Created OrderId\t\t: " + orderHolder.value.getOrderId());
if(response.getResultCode() == 400) {
for( String value : response.getValues()) {
System.out.println("Error: " + value);
}
}
env.repeatSync(orderHolder.value.getOrderId(), 10);
return response;
} catch (Exception e) {
System.out.print(e);
}
return new Response();package ascio.v2.examples;
import java.util.Calendar;
import com.ascio.www._2007._01.*;
import com.ascio.www._2007._01.holders.*;
import ascio.lib.*;
public class Transfer_DomainExample {
public static Response main() {
AscioConfig config = new AscioConfig();
V2 env = config.getTesting().getV2();
Registrant registrant = new Registrant();
registrant.setName("ascio-is-great.com");
registrant.setOrgName("Ascio");
registrant.setAddress1("Address1Test");
registrant.setAddress2("Address2Test");
registrant.setCity("CityTest");
registrant.setState("StateTest");
registrant.setPostalCode("888349");
registrant.setCountryCode("DK");
registrant.setEmail(config.getEmail());
registrant.setPhone("+45.123456789");
registrant.setFax("+45.987654321");
registrant.setRegistrantType("C1");
registrant.setVatNumber("VatNumberTest");
registrant.setNexusCategory("NexusCategoryTest");
registrant.setRegistrantNumber("abc123445");
registrant.setDetails("DetailsTest");
Contact adminContact = new Contact();
adminContact.setStatus("StatusTest");
adminContact.setHandle("JD123");
adminContact.setFirstName("John");
adminContact.setLastName("Doe");
adminContact.setOrgName("Ascio");
adminContact.setAddress1("Address1Test");
adminContact.setAddress2("Address2Test");
adminContact.setPostalCode("888349");
adminContact.setCity("CityTest");
adminContact.setState("StateTest");
adminContact.setCountryCode("DK");
adminContact.setEmail(config.getEmail());
adminContact.setPhone("+45.123456789");
adminContact.setFax("+45.987654321");
adminContact.setType("owner");
adminContact.setDetails("DetailsTest");
adminContact.setOrganisationNumber("OrganisationNumberTest");
Contact techContact = new Contact();
techContact.setStatus("StatusTest");
techContact.setHandle("JD123");
techContact.setFirstName("John");
techContact.setLastName("Doe");
techContact.setOrgName("Ascio");
techContact.setAddress1("Address1Test");
techContact.setAddress2("Address2Test");
techContact.setPostalCode("888349");
techContact.setCity("CityTest");
techContact.setState("StateTest");
techContact.setCountryCode("DK");
techContact.setEmail(config.getEmail());
techContact.setPhone("+45.123456789");
techContact.setFax("+45.987654321");
techContact.setType("owner");
techContact.setDetails("DetailsTest");
techContact.setOrganisationNumber("OrganisationNumberTest");
Contact billingContact = new Contact();
billingContact.setStatus("StatusTest");
billingContact.setHandle("JD123");
billingContact.setFirstName("John");
billingContact.setLastName("Doe");
billingContact.setOrgName("Ascio");
billingContact.setAddress1("Address1Test");
billingContact.setAddress2("Address2Test");
billingContact.setPostalCode("888349");
billingContact.setCity("CityTest");
billingContact.setState("StateTest");
billingContact.setCountryCode("DK");
billingContact.setEmail(config.getEmail());
billingContact.setPhone("+45.123456789");
billingContact.setFax("+45.987654321");
billingContact.setType("owner");
billingContact.setDetails("DetailsTest");
billingContact.setOrganisationNumber("OrganisationNumberTest");
NameServer nameServer1 = new NameServer();
nameServer1.setHostName("ns1.ascio.net");
nameServer1.setIpAddress("64.98.148.24");
nameServer1.setStatus("StatusTest");
nameServer1.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer1.setDetails("DetailsTest");
NameServer nameServer2 = new NameServer();
nameServer2.setHostName("ns2.ascio.net");
nameServer2.setIpAddress("216.40.47.100");
nameServer2.setStatus("StatusTest");
nameServer2.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer2.setDetails("DetailsTest");
NameServer nameServer3 = new NameServer();
nameServer3.setHostName("ns3.ascio.net");
nameServer3.setIpAddress("64.98.148.25");
nameServer3.setStatus("StatusTest");
nameServer3.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer3.setDetails("DetailsTest");
NameServer nameServer4 = new NameServer();
nameServer4.setHostName("ns4.ascio.net");
nameServer4.setIpAddress("216.40.47.101");
nameServer4.setStatus("StatusTest");
nameServer4.setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
nameServer4.setDetails("DetailsTest");
NameServer nameServer5 = new NameServer();
nameServer5.setHostName("HostNameTest");
nameServer5.setIpAddress("IpAddressTest");
nameServer5.setStatus("StatusTest");
nameServer5.setIpV6Address("IpV6AddressTest");
nameServer5.setDetails("DetailsTest");
NameServer nameServer6 = new NameServer();
nameServer6.setHostName("HostNameTest");
nameServer6.setIpAddress("IpAddressTest");
nameServer6.setStatus("StatusTest");
nameServer6.setIpV6Address("IpV6AddressTest");
nameServer6.setDetails("DetailsTest");
NameServer nameServer7 = new NameServer();
nameServer7.setHostName("HostNameTest");
nameServer7.setIpAddress("IpAddressTest");
nameServer7.setStatus("StatusTest");
nameServer7.setIpV6Address("IpV6AddressTest");
nameServer7.setDetails("DetailsTest");
NameServer nameServer8 = new NameServer();
nameServer8.setHostName("HostNameTest");
nameServer8.setIpAddress("IpAddressTest");
nameServer8.setStatus("StatusTest");
nameServer8.setIpV6Address("IpV6AddressTest");
nameServer8.setDetails("DetailsTest");
NameServer nameServer9 = new NameServer();
nameServer9.setHostName("HostNameTest");
nameServer9.setIpAddress("IpAddressTest");
nameServer9.setStatus("StatusTest");
nameServer9.setIpV6Address("IpV6AddressTest");
nameServer9.setDetails("DetailsTest");
NameServer nameServer10 = new NameServer();
nameServer10.setHostName("HostNameTest");
nameServer10.setIpAddress("IpAddressTest");
nameServer10.setStatus("StatusTest");
nameServer10.setIpV6Address("IpV6AddressTest");
nameServer10.setDetails("DetailsTest");
NameServer nameServer11 = new NameServer();
nameServer11.setHostName("HostNameTest");
nameServer11.setIpAddress("IpAddressTest");
nameServer11.setStatus("StatusTest");
nameServer11.setIpV6Address("IpV6AddressTest");
nameServer11.setDetails("DetailsTest");
NameServer nameServer12 = new NameServer();
nameServer12.setHostName("HostNameTest");
nameServer12.setIpAddress("IpAddressTest");
nameServer12.setStatus("StatusTest");
nameServer12.setIpV6Address("IpV6AddressTest");
nameServer12.setDetails("DetailsTest");
NameServers nameServers = new NameServers();
nameServers.setNameServer1(nameServer1);
nameServers.setNameServer2(nameServer2);
nameServers.setNameServer3(nameServer3);
nameServers.setNameServer4(nameServer4);
nameServers.setNameServer5(nameServer5);
nameServers.setNameServer6(nameServer6);
nameServers.setNameServer7(nameServer7);
nameServers.setNameServer8(nameServer8);
nameServers.setNameServer9(nameServer9);
nameServers.setNameServer10(nameServer10);
nameServers.setNameServer11(nameServer11);
nameServers.setNameServer12(nameServer12);
TradeMark trademark = new TradeMark();
trademark.setName("ascio-is-great.com");
trademark.setCountry("DK");
trademark.setDate(Calendar.getInstance());
trademark.setNumber("NumberTest");
trademark.setType("owner");
trademark.setContact("ContactTest");
trademark.setContactLanguage("ContactLanguageTest");
trademark.setDocumentationLanguage("DocumentationLanguageTest");
trademark.setSecondContact("SecondContactTest");
trademark.setThirdContact("ThirdContactTest");
trademark.setRegDate(Calendar.getInstance());
DnsSecKey dnsSecKey1 = new DnsSecKey();
dnsSecKey1.setHandle("JD123");
DnsSecKey dnsSecKey2 = new DnsSecKey();
dnsSecKey2.setHandle("JD123");
DnsSecKey dnsSecKey3 = new DnsSecKey();
dnsSecKey3.setHandle("JD123");
DnsSecKey dnsSecKey4 = new DnsSecKey();
dnsSecKey4.setHandle("JD123");
DnsSecKey dnsSecKey5 = new DnsSecKey();
dnsSecKey5.setHandle("JD123");
DnsSecKeys dnsSecKeys = new DnsSecKeys();
dnsSecKeys.setDnsSecKey1(dnsSecKey1);
dnsSecKeys.setDnsSecKey2(dnsSecKey2);
dnsSecKeys.setDnsSecKey3(dnsSecKey3);
dnsSecKeys.setDnsSecKey4(dnsSecKey4);
dnsSecKeys.setDnsSecKey5(dnsSecKey5);
Extension extension = new Extension();
extension.setKey("Title");
extension.setValue("Mr.");
ExtensionsExtension extensions[] = new ExtensionsExtension[]{extension};
PrivacyProxy privacyProxy = new PrivacyProxy();
privacyProxy.setType(PrivacyProxyType.None);
privacyProxy.setPrivacyAdmin(false);
privacyProxy.setPrivacyTech(false);
privacyProxy.setPrivacyBilling(false);
privacyProxy.setExtensions(extensions);
Domain domain = new Domain();
domain.setDomainName(env.getProperties().getProperty("DomainName"));
domain.setAuthInfo("X4FF!zu");
domain.setRegistrant(registrant);
domain.setAdminContact(adminContact);
domain.setTechContact(techContact);
domain.setBillingContact(billingContact);
domain.setNameServers(nameServers);
domain.setTrademark(trademark);
domain.setDnsSecKeys(dnsSecKeys);
domain.setPrivacyProxy(privacyProxy);
domain.setDiscloseSocialData("true");
Order order = new Order();
order.setType(OrderType.Transfer_Domain);
order.setAccountReference("AccountReferenceTest");
order.setTransactionComment("TransactionCommentTest");
order.setComments("RegistrarTag");
order.setOptions("OptionsTest");
order.setLocalPresence("off");
order.setBatch("BatchTest");
order.setDocumentation("DocumentationTest");
order.setDomain(domain);
order.setAgreedPrice(5000);
try {
/**inputs holders*/
OrderHolder orderHolder = new OrderHolder(order);
Response response = env.getClient().createOrder(env.getSessionId(), orderHolder);
System.out.println("CreateOrder ResultCode\t: " + response.getResultCode().toString());
System.out.println("CreateOrder Message\t: " + response.getMessage());
System.out.println("Created OrderId\t\t: " + orderHolder.value.getOrderId());
if(response.getResultCode() == 400) {
for( String value : response.getValues()) {
System.out.println("Error: " + value);
}
}
env.repeatSync(orderHolder.value.getOrderId(), 10);
return response;
} catch (Exception e) {
System.out.print(e);
}
return new Response();
WSDL for AWS v2
https://aws.demo.ascio.com/2012/01/01/AscioService.wsdl (OTE)
https://aws.ascio.com/2012/01/01/AscioService.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.
https://aws.demo.ascio.com/2012/01/01/AscioService.wsdl (OTE)
https://aws.ascio.com/2012/01/01/AscioService.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.