Ascio Web Service v2
PHP 5 Classes CreateOrder - Nameserver_Update
Replaces the nameserver with a new handle. There are 2 ways to submit the new Nameserver: With the Nameserver Update, it is possible to change Tech Contact, DnsSec and Nameservers in one request. For .com, .net and some other TLDs glue records are needed. Please contact support for help.
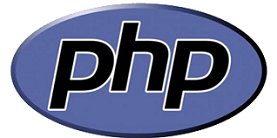
Response CreateOrder(string $sessionId,Order $order)
Response codes
ResultCode | Message | Value |
---|---|---|
200 | Order validated | |
200 | Order received | |
400 | Order not validated | Messages |
401 | Authorization failed | |
405 | Access denied |
CreateOrder Request
Property | Description |
---|---|
sessionId | Example: o58t9fjgw9bjarp6q7byv13e |
order |
CreateOrderResponse
Property |
---|
CreateOrderResult |
order |
Property | Value |
OrderType | Nameserver_Update |
---|
PHP 5 Classes example
<?php
namespace ascio\v2\examples;
require(__DIR__."/../service/autoload.php");
require(__DIR__."/../../lib/AscioConfig.php");
use ascio\v2 as ascio;
use ascio\lib as lib;
function nameserver_UpdateExample() {
$config = new lib\Config();
$env = $config->get("testing"); //testing or live
$sessionId = "12345";
$ascioClient = new ascio\AscioServices(array("trace" => true),$env->getWsdl("v2"));
$techContact = new ascio\Contact();
$techContact->setStatus("StatusTest");
$techContact->setHandle("JD123");
$techContact->setFirstName("John");
$techContact->setLastName("Doe");
$techContact->setOrgName("Ascio");
$techContact->setAddress1("Address1Test");
$techContact->setAddress2("Address2Test");
$techContact->setPostalCode("888349");
$techContact->setCity("CityTest");
$techContact->setState("StateTest");
$techContact->setCountryCode("DK");
$techContact->setEmail($config->getEmail());
$techContact->setPhone("+45.123456789");
$techContact->setFax("+45.987654321");
$techContact->setType("owner");
$techContact->setDetails("DetailsTest");
$techContact->setOrganisationNumber("OrganisationNumberTest");
$nameServer1 = new ascio\NameServer();
$nameServer1->setHostName("ns1.ascio.net");
$nameServer1->setIpAddress("64.98.148.24");
$nameServer1->setStatus("StatusTest");
$nameServer1->setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
$nameServer1->setDetails("DetailsTest");
$nameServer2 = new ascio\NameServer();
$nameServer2->setHostName("ns2.ascio.net");
$nameServer2->setIpAddress("216.40.47.100");
$nameServer2->setStatus("StatusTest");
$nameServer2->setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
$nameServer2->setDetails("DetailsTest");
$nameServer3 = new ascio\NameServer();
$nameServer3->setHostName("ns3.ascio.net");
$nameServer3->setIpAddress("64.98.148.25");
$nameServer3->setStatus("StatusTest");
$nameServer3->setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
$nameServer3->setDetails("DetailsTest");
$nameServer4 = new ascio\NameServer();
$nameServer4->setHostName("ns4.ascio.net");
$nameServer4->setIpAddress("216.40.47.101");
$nameServer4->setStatus("StatusTest");
$nameServer4->setIpV6Address("xxxx:xxxx:xxx:xxx:xxxx");
$nameServer4->setDetails("DetailsTest");
$nameServer5 = new ascio\NameServer();
$nameServer5->setHostName("HostNameTest");
$nameServer5->setIpAddress("IpAddressTest");
$nameServer5->setStatus("StatusTest");
$nameServer5->setIpV6Address("IpV6AddressTest");
$nameServer5->setDetails("DetailsTest");
$nameServer6 = new ascio\NameServer();
$nameServer6->setHostName("HostNameTest");
$nameServer6->setIpAddress("IpAddressTest");
$nameServer6->setStatus("StatusTest");
$nameServer6->setIpV6Address("IpV6AddressTest");
$nameServer6->setDetails("DetailsTest");
$nameServer7 = new ascio\NameServer();
$nameServer7->setHostName("HostNameTest");
$nameServer7->setIpAddress("IpAddressTest");
$nameServer7->setStatus("StatusTest");
$nameServer7->setIpV6Address("IpV6AddressTest");
$nameServer7->setDetails("DetailsTest");
$nameServer8 = new ascio\NameServer();
$nameServer8->setHostName("HostNameTest");
$nameServer8->setIpAddress("IpAddressTest");
$nameServer8->setStatus("StatusTest");
$nameServer8->setIpV6Address("IpV6AddressTest");
$nameServer8->setDetails("DetailsTest");
$nameServer9 = new ascio\NameServer();
$nameServer9->setHostName("HostNameTest");
$nameServer9->setIpAddress("IpAddressTest");
$nameServer9->setStatus("StatusTest");
$nameServer9->setIpV6Address("IpV6AddressTest");
$nameServer9->setDetails("DetailsTest");
$nameServer10 = new ascio\NameServer();
$nameServer10->setHostName("HostNameTest");
$nameServer10->setIpAddress("IpAddressTest");
$nameServer10->setStatus("StatusTest");
$nameServer10->setIpV6Address("IpV6AddressTest");
$nameServer10->setDetails("DetailsTest");
$nameServer11 = new ascio\NameServer();
$nameServer11->setHostName("HostNameTest");
$nameServer11->setIpAddress("IpAddressTest");
$nameServer11->setStatus("StatusTest");
$nameServer11->setIpV6Address("IpV6AddressTest");
$nameServer11->setDetails("DetailsTest");
$nameServer12 = new ascio\NameServer();
$nameServer12->setHostName("HostNameTest");
$nameServer12->setIpAddress("IpAddressTest");
$nameServer12->setStatus("StatusTest");
$nameServer12->setIpV6Address("IpV6AddressTest");
$nameServer12->setDetails("DetailsTest");
$nameServers = new ascio\NameServers();
$nameServers->setNameServer1($nameServer1);
$nameServers->setNameServer2($nameServer2);
$nameServers->setNameServer3($nameServer3);
$nameServers->setNameServer4($nameServer4);
$nameServers->setNameServer5($nameServer5);
$nameServers->setNameServer6($nameServer6);
$nameServers->setNameServer7($nameServer7);
$nameServers->setNameServer8($nameServer8);
$nameServers->setNameServer9($nameServer9);
$nameServers->setNameServer10($nameServer10);
$nameServers->setNameServer11($nameServer11);
$nameServers->setNameServer12($nameServer12);
$dnsSecKey1 = new ascio\DnsSecKey();
$dnsSecKey1->setHandle("JD123");
$dnsSecKey2 = new ascio\DnsSecKey();
$dnsSecKey2->setHandle("JD123");
$dnsSecKey3 = new ascio\DnsSecKey();
$dnsSecKey3->setHandle("JD123");
$dnsSecKey4 = new ascio\DnsSecKey();
$dnsSecKey4->setHandle("JD123");
$dnsSecKey5 = new ascio\DnsSecKey();
$dnsSecKey5->setHandle("JD123");
$dnsSecKeys = new ascio\DnsSecKeys();
$dnsSecKeys->setDnsSecKey1($dnsSecKey1);
$dnsSecKeys->setDnsSecKey2($dnsSecKey2);
$dnsSecKeys->setDnsSecKey3($dnsSecKey3);
$dnsSecKeys->setDnsSecKey4($dnsSecKey4);
$dnsSecKeys->setDnsSecKey5($dnsSecKey5);
$domain = new ascio\Domain();
$domain->setDomainName($env->getProperties()->getProperty("DomainName"));
$domain->setTechContact($techContact);
$domain->setNameServers($nameServers);
$domain->setDnsSecKeys($dnsSecKeys);
$order = new ascio\Order();
$order->setType(ascio\OrderType::Nameserver_Update);
$order->setDomain($domain);
echo "start validate\n";
$validateOrder = new ascio\ValidateOrder($sessionId, $order);
try {
$response = $ascioClient->ValidateOrder($validateOrder);
} catch (\Exception $e) {
echo ("[".$e->faultcode . "] ". $e->faultstring);
return;
}
echo "end validate\n";
if ($response->getValidateOrderResult()->getResultCode() == 200) {
echo "Validation: OK\r\n";
try {
$createOrderResponse = $ascioClient->CreateOrder(new ascio\CreateOrder($sessionId, $order));
} catch (\Exception $e) {
echo ("[".$e->faultcode . "] ". $e->faultstring);
return;
}
echo "Create Order: ".$createOrderResponse->CreateOrderResult->getResultCode() . " " . $createOrderResponse->CreateOrderResult->getResultMessage() . "\r\n";
if ($createOrderResponse->CreateOrderResult->getResultCode() == 200) {
echo "OrderId : " . $createOrderResponse->CreateOrderResult->getOrderInfo()->getOrderId() . "\r\n";
echo "Order Status : " . $createOrderResponse->CreateOrderResult->getOrderInfo()->getStatus() . "\r\n";
} else {
echo ($response->CreateOrderResult->getMessage()."\n");
foreach($createOrderResponse->CreateOrderResult->getErrors() as $error) {
echo $error."\n";
return $response;
}
}
return $createOrderResponse;
} else {
echo ($response->getValidateOrderResult()->getMessage()."\n");
if ($response->getValidateOrderResult()->getErrors()) {
foreach($response->getValidateOrderResult()->getErrors()->getString() as $error) {
echo $error."\n";
}
}
return $response;
}
}
nameserver_UpdateExample();
WSDL for AWS v2
https://aws.demo.ascio.com/2012/01/01/AscioService.wsdl (OTE)
https://aws.ascio.com/2012/01/01/AscioService.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.
https://aws.demo.ascio.com/2012/01/01/AscioService.wsdl (OTE)
https://aws.ascio.com/2012/01/01/AscioService.wsdl (Live)
Please configure the IP-Whitelisting in the portal/demo-portal.